Looping through rows and columns #270
Labels
No Label
DBF
Dates
Defined Names
Features
Formula
HTML
Images
Infrastructure
Integration
International
ODS
Operations
Performance
PivotTables
Pro
Protection
Read Bug
SSF
SYLK
Style
Write Bug
good first issue
No Milestone
No Assignees
1 Participants
Notifications
Due Date
No due date set.
Dependencies
No dependencies set.
Reference: sheetjs/sheetjs#270
Loading…
Reference in New Issue
No description provided.
Delete Branch "%!s(<nil>)"
Deleting a branch is permanent. Although the deleted branch may continue to exist for a short time before it actually gets removed, it CANNOT be undone in most cases. Continue?
How do I loop through rows and columns without converting the worksheet to csv or json before doing so?
Is it not possible to do something like
Convert to an array:
Then loop through that array.
@Mithgol, I seem to not have sheet['!range'] in xlsx files. Is there a new way to get the ranges? (This function works fine for xls files)
@gregpinero @Mithgol the
!ref
key is exposed by every format.!range
was used in XLS but it was ultimately confusing to store the same information in 2 different ways. Decoding usingdecode_range
gives you the range object. So @Mithgol 's code sample would look likeIs it worth adding an "iterator" to the utils? Basically it would take a worksheet and a callback function and repeatedly call it for every cell in the workbook, with an option to include or omit empty cells
Thanks. That does work.
I would like something just like sheet2arr above in the utils. That what I thought XLSX.utils.sheet_to_row_object_array would do but it seemed to give me strange results missing most of the data.
@gregpinero the JSON conversion has a little bit of "intelligence" and is poorly documented :/
For example consider this worksheet:
Let's say we are in node (the relevant parts work the same way in browser). Read the workbook:
So by default, the conversion will read the first row and use them as keys in the row object:
If a field is missing, the data won't show up. If there's data in a cell with no header (say, data in cell D2 but not D1), data won't show up.
passing an options argument with
header
controls the output:Setting header to
1
will generate arrays:Setting header to
A
will use the column names:You can even give custom labels by passing an array:
The easiest way to control the range is to change the
!ref
value on the sheet. It's expected to be an A1-style range like "A1:C4". Changing the value will change the range that the utils look at:The same parameter also affects writing workbooks -- cells outside of that range are not included:
Thanks, that makes a lot more sense now. My case was weird because I have the data I want starting at row 5. But I guess I could have manually given it a header and ignore data before row 4. I'll give that a try new time.
@gregpinero @mazing @Mithgol we improved the README a bit: https://github.com/SheetJS/js-xlsx#json -- can you take a look when you get a chance?
Closing for now, please follow up with some feedback on the relevant documentation if you get a chance :D
HI My requirement is like this ,how can i achieve this?
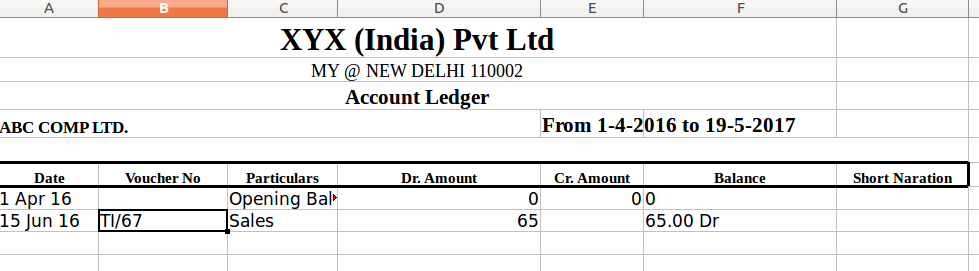
I dnt know
Hello...I have one query ..actually I want to display excel file in select box in html and I'm done with this but after that,when we select option from select box that time i wan to display other information from that excel file..how can I do this...
I know I'm late but it might help other having same requirement.
You can do that by specifying range attribute like this
var sheetName = workbook.SheetNames[0]; var d = XLSX.utils.sheet_to_json(workbook.Sheets[sheetName], { range: 5 });
And it will return data for the table in d after row 5.
Thanks
Hi,
I have exported json to excel using XLSX.utils.sheet_to_json and the output looks like below. I would like to display 'Total' and its contents as the last column. How can I achieve that? Please note that I cannot order all the columns as some columns are dynamic and varies from one situation to another. For the common ones, I have added header order. My requirement is to display the below headers first, then the dynamic ones followed by total. Total should always be in the end.
const headerInfo = ['Event Type', 'Start Date', 'End Date', 'Duration Name'];
const worksheet: XLSX.WorkSheet = XLSX.utils.json_to_sheet(json, { header: headerInfo });
Try this code, read the properties of first object in json array and create add it into headerInfo array and lastly add Total.
var headerInfo = ['Event Type', 'Start Date', 'End Date', 'Duration Name']; for (var key in json[0]) { if (headerInfo.indexOf(key) == -1 && key !='Total') headerInfo.push(key); } headerInfo.push('Total'); const worksheet: XLSX.WorkSheet = XLSX.utils.json_to_sheet(json, { header: headerInfo });