Unable to format date to dd-mm-yyyy while using XLSX.utils.json_to_sheet #2919
Labels
No Label
DBF
Dates
Defined Names
Features
Formula
HTML
Images
Infrastructure
Integration
International
ODS
Operations
Performance
PivotTables
Pro
Protection
Read Bug
SSF
SYLK
Style
Write Bug
good first issue
No Milestone
No Assignees
2 Participants
Notifications
Due Date
No due date set.
Dependencies
No dependencies set.
Reference: sheetjs/sheetjs#2919
Loading…
Reference in New Issue
No description provided.
Delete Branch "%!s(<nil>)"
Deleting a branch is permanent. Although the deleted branch may continue to exist for a short time before it actually gets removed, it CANNOT be undone in most cases. Continue?
This is my data
I am trying to format dates(i.e. date1 ) in such a way that when I view these dates in excel they should be visible like:


But when I click to edit the cells. These dates should be like:
05-04-2023
08-04-2023
Here is the screenshot of what I want:
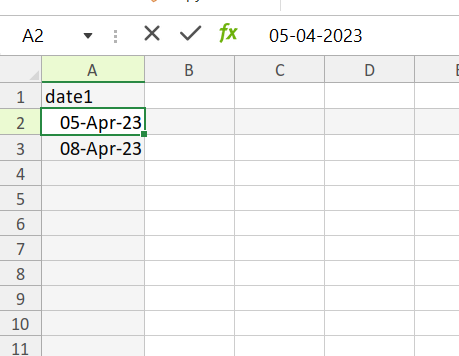
Here is the screenshot of what I am getting as output:
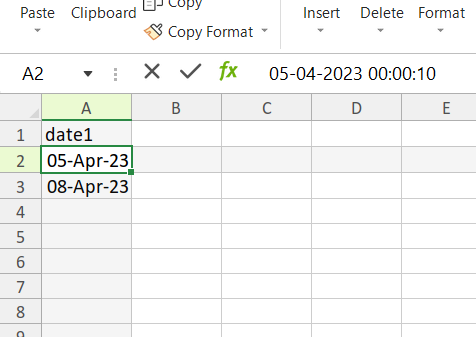
When I am trying to edit a cell I am also getting hh:mm:ss component of date (05-04-2023 00:00:10 00:00:10 is the component I do not want).
Here is my code converting date to excel:
How can I remove time compoent from the date in the output excel sheet?
Date handling has been overhauled in 0.20.0.
To generate the desired sheet: https://jsfiddle.net/wgtacevz/
If you are modifying the worksheet object directly, the dates that Excel will show in the formula bar are the dates as interpreted using the UTC methods. That means
date.getUTCHours()
,date.getUTCMinutes()
,date.getUTCSeconds()
anddate.getUTCMilliseconds()
must all be0
.If you are using
json_to_sheet
oraoa_to_sheet
, theUTC
parameter controls whether to interpret the dates using UTC methods or using local methods.https://docs.sheetjs.com/docs/csf/features/dates explains in more detail.