How to get colums instead of rows #3114
Labels
No Label
DBF
Dates
Defined Names
Features
Formula
HTML
Images
Infrastructure
Integration
International
ODS
Operations
Performance
PivotTables
Pro
Protection
Read Bug
SSF
SYLK
Style
Write Bug
good first issue
No Milestone
No Assignees
2 Participants
Notifications
Due Date
No due date set.
Dependencies
No dependencies set.
Reference: sheetjs/sheetjs#3114
Loading…
Reference in New Issue
Block a user
No description provided.
Delete Branch "%!s()"
Deleting a branch is permanent. Although the deleted branch may continue to exist for a short time before it actually gets removed, it CANNOT be undone in most cases. Continue?
Something like
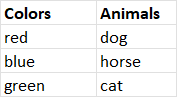
Transposed to something like
or
You can manually transpose the data. https://docs.sheetjs.com/docs/demos/math/tensorflow#exporting-datasets-to-a-worksheet shows an example that creates a copy of the array of arrays:
This gives you the second form. The first form can be recovered with
Object.fromEntries
:Thanks, that worked!
I also rewrote it with
map
instead offor
.Returning

Perhaps add a transpose option to support it natively?