.. | ||
src | ||
versions | ||
.angular-cli.json | ||
.eslintrc | ||
.gitattributes | ||
.gitignore | ||
ionic-app.module.ts | ||
ionic.sh | ||
ionic.ts | ||
Makefile | ||
nscript.sh | ||
nscript.ts | ||
package.json | ||
README.md | ||
screen.png | ||
tsconfig.json |
Angular 2+
The library can be imported directly from TS code with:
import * as XLSX from 'xlsx';
This demo uses an array of arrays (type Array<Array<any>>
) as the core state.
The component template includes a file input element, a table that updates with
the data, and a button to export the data.
Other scripts in this demo show:
ionic
deployment for iOS, android, and browsernativescript
deployment for iOS and android
Array of Arrays
Array<Array<any>>
neatly maps to a table with ngFor
:
<table class="sjs-table">
<tr *ngFor="let row of data">
<td *ngFor="let val of row">
{{val}}
</td>
</tr>
</table>
The aoa_to_sheet
utility function returns a worksheet. Exporting is simple:
/* generate worksheet */
const ws: XLSX.WorkSheet = XLSX.utils.aoa_to_sheet(this.data);
/* generate workbook and add the worksheet */
const wb: XLSX.WorkBook = XLSX.utils.book_new();
XLSX.utils.book_append_sheet(wb, ws, 'Sheet1');
/* save to file */
XLSX.writeFile(wb, 'SheetJS.xlsx');
sheet_to_json
with the option header:1
makes importing simple:
/* <input type="file" (change)="onFileChange($event)" multiple="false" /> */
/* ... (within the component class definition) ... */
onFileChange(evt: any) {
/* wire up file reader */
const target: DataTransfer = <DataTransfer>(evt.target);
if (target.files.length !== 1) throw new Error('Cannot use multiple files');
const reader: FileReader = new FileReader();
reader.onload = (e: any) => {
/* read workbook */
const ab: ArrayBuffer = e.target.result;
const wb: XLSX.WorkBook = XLSX.read(ab);
/* grab first sheet */
const wsname: string = wb.SheetNames[0];
const ws: XLSX.WorkSheet = wb.Sheets[wsname];
/* save data */
this.data = <AOA>(XLSX.utils.sheet_to_json(ws, {header: 1}));
};
reader.readAsArrayBuffer(target.files[0]);
}
Switching between Angular versions
Modules that work with Angular 2 largely work as-is with Angular 4+. Switching
between versions is mostly a matter of installing the correct version of the
core and associated modules. This demo includes package.json-angular#
files
for every major version of Angular up to 12.
To test a particular Angular version, overwrite package.json
:
# switch to Angular 2
$ cp package.json-ng2 package.json
$ npm install
$ ng serve
Note: when running the demos, Angular 2 requires Node <= 14. This is due to a
tooling issue with ng
and does not affect browser use.
XLSX Symbolic Link
In this tree, node_modules/xlsx
is a link pointing back to the root. This
enables testing the development version of the library. In order to use this
demo in other applications, add the xlsx
dependency:
$ npm install --save xlsx
SystemJS Configuration
The default angular-cli configuration requires no additional configuration.
Some deployments use the SystemJS loader, which does require configuration. The SystemJS example shows the required meta and map settings:
SystemJS.config({
meta: {
'xlsx': {
exports: 'XLSX' // <-- tell SystemJS to expose the XLSX variable
}
},
map: {
'xlsx': 'xlsx.full.min.js', // <-- make sure xlsx.full.min.js is in same dir
'fs': '', // <--|
'crypto': '', // <--| suppress native node modules
'stream': '' // <--|
}
});
Ionic
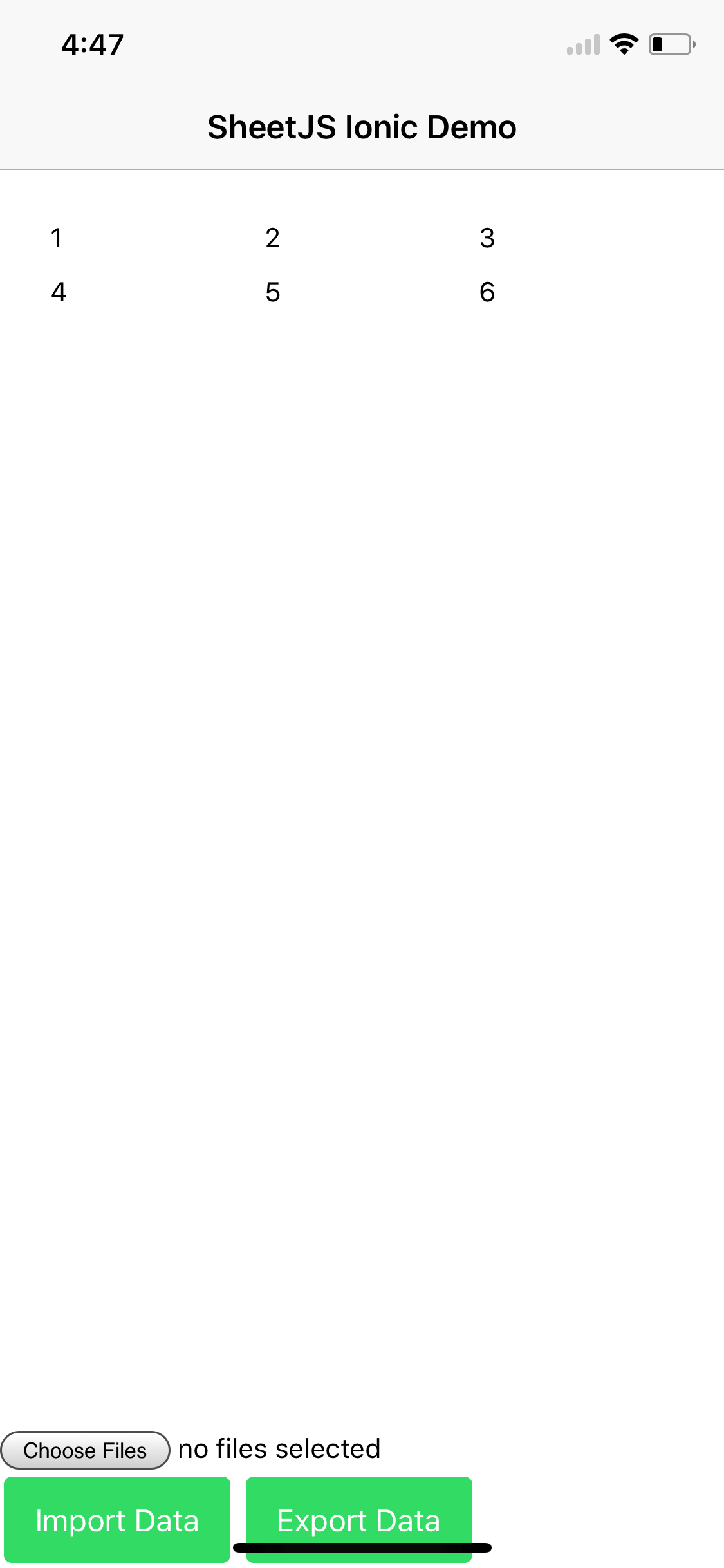
Reproducing the full project is a little bit tricky. The included ionic.sh
script performs the necessary installation steps.
Array<Array<any>>
neatly maps to a table with ngFor
:
<ion-grid>
<ion-row *ngFor="let row of data">
<ion-col *ngFor="let val of row">
{{val}}
</ion-col>
</ion-row>
</ion-grid>
@ionic-native/file
reads and writes files on devices. readAsArrayBuffer
returns ArrayBuffer
objects suitable for array
type, and array
type can
be converted to blobs that can be exported with writeFile
:
/* read a workbook */
const ab: ArrayBuffer = await this.file.readAsArrayBuffer(url, filename);
const wb: XLSX.WorkBook = XLSX.read(bstr, {type: 'array'});
/* write a workbook */
const wbout: ArrayBuffer = XLSX.write(wb, { bookType: 'xlsx', type: 'array' });
let blob = new Blob([wbout], {type: 'application/octet-stream'});
this.file.writeFile(url, filename, blob, {replace: true});
NativeScript
Reproducing the full project is a little bit tricky. The included nscript.sh
script performs the necessary installation steps and adds the necessary shims
for async
support. Due to incompatibilities with NativeScript and TypeScript
definitions, apps should require the xlsx.full.min.js
file directly:
const XLSX = require("./xlsx.full.min.js");
The ISO_8859_1
encoding from the text module specifies "binary"
strings.
File#readText
and File#writeText
reads and writes files:
/* read a workbook */
const bstr: string = await file.readText(encoding.ISO_8859_1);
const wb = XLSX.read(bstr, { type: "binary" });
/* write a workbook */
const wbout: string = XLSX.write(wb, { bookType: 'xlsx', type: 'binary' });
await file.writeText(wbout, encoding.ISO_8859_1);
Note: some versions of NativeScript do not properly support typed arrays or binary strings. See https://github.com/NativeScript/NativeScript/issues/9586